Mastering String Formatting in JavaScript with sprintf()
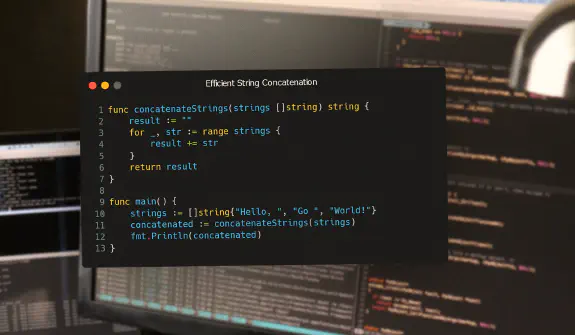
String formatting is a fundamental aspect of programming, allowing developers to create dynamic and readable text output by embedding variables and applying specific formatting rules. The sprintf function, originating from the C programming language, has become a standard for this purpose across numerous languages. It provides a powerful and structured way to construct strings by specifying placeholders that are then replaced with provided values.
While JavaScript doesn't have a built-in sprintf function, libraries like Playful Sparkle's sprintf.js (opens in new window) bridge this gap. This lightweight and efficient library brings the familiar and robust sprintf functionality to the JavaScript ecosystem.
Here's a simple example of how `sprintf` works in general:
// General concept (not JavaScript's native)
const name = "Alice";
const age = 30;
const message = sprintf("My name is %s and I am %d years old.", name, age);
// Expected output: "My name is Alice and I am 30 years old."
TL;DR
This article explores the power of string formatting in JavaScript using the sprintf
method and the sprintf.js library developed by Playful Sparkle. It highlights the advantages of sprintf over simple string concatenation, delves into the library's API and features, provides practical use cases, and offers guidance on its application in your projects. If you need precise control over string output, improved readability, and a familiar formatting style, sprintf.js is a valuable tool.
The Ubiquity of sprintf
The sprintf
paradigm, or functions with similar syntax and capabilities, is prevalent in many programming languages. Developers familiar with languages like C, Python (%
operator or f-strings with similar formatting options), PHP (sprintf
), Java (String.format
), and many others will recognize the power and convenience of this approach.
For instance, in Python, you might write:
name = "Bob"
price = 99.99
message = "The product '%s' costs %.2f." % (name, price)
print(message) # Output: The product 'Bob' costs 99.99.
JavaScript, however, lacks a direct equivalent built into the language. This is where libraries like sprintf.js become essential, offering JavaScript developers the same level of control and expressiveness for string formatting.
Why sprintf is Better Than String Concatenation
Traditional string concatenation in JavaScript often involves using the +
operator or template literals. While these methods work for simple cases, they can become cumbersome and less readable for more complex formatting requirements. sprintf
offers several advantages:
Readability: When dealing with multiple variables and formatting requirements, sprintf
keeps the string template and the values separate, making the code easier to read and understand.
// String concatenation
const itemName = "Laptop";
const discount = 0.15;
const price = 1200;
const messageConcat = "The " + itemName + " is on sale! You save " + (discount * 100) + "% and pay $" + (price * (1 - discount)).toFixed(2) + ".";
console.log(messageConcat);
// Using sprintf.js
import { sprintf } from '@playfulsparkle/sprintf-js';
const messageSprintf = sprintf("The %s is on sale! You save %.0f%% and pay $%.2f.", itemName, discount * 100, price * (1 - discount));
console.log(messageSprintf);
As you can see, the sprintf
version is significantly more concise and clearly outlines the structure of the final string.
Control Over Formatting: sprintf
provides precise control over how values are formatted, including:
- Padding: Adding characters (like spaces or zeros) to ensure a certain width.
- Alignment: Left, right, or center alignment within the specified width.
- Precision: Controlling the number of decimal places for floating-point numbers.
import { sprintf } from '@playfulsparkle/sprintf-js';
const num = 12.345;
const paddedNum = sprintf("%05.2f", num); // Output: "012.35" (padded with leading zeros, 2 decimal places)
const alignedText = sprintf("%10s", "Hello"); // Output: " Hello" (right-aligned, width 10)
Suitability for Localization: When preparing strings for different languages, sprintf
-like formats often integrate well with localization frameworks. The separation of the string template from the actual values makes it easier to manage translations. While sprintf.js itself doesn't handle full internationalization (like pluralization rules), it's excellent for formatting the data within translated strings.
Introducing sprintf.js
Playful Sparkle's sprintf.js is a well-maintained, lightweight, and open-source library that faithfully implements the sprintf
functionality in JavaScript. It's a fantastic choice for developers who need robust string formatting capabilities without the overhead of larger utility libraries.
Key Features
- Faithful Implementation: Adheres closely to the standard sprintf behavior found in other languages.
- Lightweight: Has a minimal footprint, ensuring it doesn't significantly impact your application's size.
- Open Source: Freely available under a permissive license, allowing for use in various projects.
Installation
You can easily install sprintf.js using either NPM or Bower:
NPM:
npm install @playfulsparkle/sprintf-js
Bower:
bower install playfulsparkle/sprintf.js
Basic Installation Example (using NPM in a Node.js environment)
// Import the sprintf function
import { sprintf } from '@playfulsparkle/sprintf-js';
const greeting = sprintf("Hello, %s!", "World");
console.log(greeting); // Output: Hello, World!
Diving into the sprintf.js API
sprintf.js primarily offers two functions: sprintf()
and vsprintf()
.
string sprintf(string format, mixed arg1?, mixed arg2?, ...)
This is the main function. It takes a format string as the first argument, followed by the values to be inserted into the string according to the placeholders in the format string.
import { sprintf } from '@playfulsparkle/sprintf-js';
const name = "Charlie";
const score = 95;
const message = sprintf("Student %s achieved a score of %d.", name, score);
console.log(message); // Output: Student Charlie achieved a score of 95.
const pi = Math.PI;
const formattedPi = sprintf("The value of pi is approximately %.2f.", pi);
console.log(formattedPi); // Output: The value of pi is approximately 3.14.
string vsprintf(string format, array argv)
This function is similar to sprintf()
, but it accepts an array of arguments instead of individual arguments. This is particularly useful when you have the values to be formatted stored in an array.
import { vsprintf } from '@playfulsparkle/sprintf-js';
const data = ["David", 88, "Science"];
const message = vsprintf("Student %s scored %d in %s.", data);
console.log(message); // Output: Student David scored 88 in Science.
Mastering Format String Placeholders
The power of sprintf.js lies in its format string placeholders. These placeholders define how the provided arguments should be formatted and inserted into the resulting string. The general syntax for a placeholder is:
%[argument_index$][flags][width][.precision]type
Let's break down each component:
%
: The literal percent sign that marks the beginning of a placeholder.[argument_index$]
(Optional): Allows you to explicitly specify which argument should be used for this placeholder. For example,%2$s
would use the second argument as a string.[flags]
(Optional): Modifiers that affect the output format. Common flags include:+
: Forces to precede the result with a plus or minus sign on numeric types.: (space) If no sign is going to be written, a blank space is inserted before the number.
0
: Pads the number with leading zeros to the specified width.-
: Left-justifies the result within the specified width.
[width]
(Optional): An integer that specifies the minimum number of characters to be output. If the output is shorter than this number, it will be padded (usually with spaces, or zeros if the0
flag is used).[.precision]
(Optional): Specifies the number of digits after the decimal point for floating-point numbers, or the maximum length of a string. It's preceded by a dot (.
).type
(Required): A single character that specifies the type of the argument to be formatted. Here's a table of common type specifiers supported by sprintf.js:
Type Specifier | Description | Example | Output |
---|---|---|---|
% | A literal percent sign. No argument is consumed. | sprintf("%%") | % |
b | Argument is treated as an integer and presented as a binary number. | sprintf("%b", 10) | 1010 |
c | Argument is treated as an integer and presented as the character with that ASCII value. | sprintf("%c", 65) | A |
d or i | Argument is treated as an integer and presented as a signed decimal number. | sprintf("%d", 123) | 123 |
e | Argument is treated as a float and presented in scientific notation (e.g., 1.23e+2). | sprintf("%e", 123.45) | 1.234500e+2 |
E | Same as e but uses uppercase letter (e.g., 1.23E+2). | sprintf("%E", 123.45) | 1.234500E+2 |
f | Argument is treated as a float and presented as a decimal number. | sprintf("%f", 12.345) | 12.345000 |
F | Same as f . | sprintf("%F", 12.345) | 12.345000 |
g | Shorter of %e and %f . | sprintf("%g", 123.45) | 123.45 |
G | Shorter of %E and %F . | sprintf("%G", 123.45) | 123.45 |
o | Argument is treated as an integer and presented as an octal number. | sprintf("%o", 10) | 12 |
s | Argument is treated as and presented as a string. | sprintf("%s", "Hello") | Hello |
t | Argument is treated as a boolean and presented as "true" or "false". | sprintf("%t", true) | true |
T | Same as t but uses uppercase letters ("TRUE" or "FALSE"). | sprintf("%T", false) | FALSE |
u | Argument is treated as an integer and presented as an unsigned decimal number. | sprintf("%u", -10) | 4294967286 |
x | Argument is treated as an integer and presented as a hexadecimal number (lowercase). | sprintf("%x", 255) | ff |
X | Same as x but uses uppercase letters. | sprintf("%X", 255) | FF |
Illustrative Code Examples
import { sprintf } from '@playfulsparkle/sprintf-js';
// Argument Index
console.log(sprintf("%2$s %1$s", "World", "Hello")); // Output: Hello World
// Flags
console.log(sprintf("%+d", 10)); // Output: +10
console.log(sprintf("% d", 10)); // Output: 10
console.log(sprintf("%05d", 5)); // Output: 00005
console.log(sprintf("%-5s", "Hi")); // Output: Hi
// Width
console.log(sprintf("%10s", "Test")); // Output: Test (right-aligned)
console.log(sprintf("%-10s", "Test")); // Output: Test (left-aligned)
// Precision
console.log(sprintf("%.2f", 3.14159)); // Output: 3.14
console.log(sprintf("%.5s", "JavaScript")); // Output: JavaS
// Combining options
console.log(sprintf("%010.2f", 123.456)); // Output: 0000123.46
Unleashing Advanced Features of sprintf.js
sprintf.js offers some advanced features that can further enhance your string formatting capabilities.
Flexible Argument Order
As seen in the "Argument Index" example above, you can explicitly specify the order in which arguments are used by adding n$
after the %
in the placeholder, where n
is the index of the argument (starting from 1). This is particularly useful when you need to reuse arguments or when the order of arguments in your code doesn't match the desired output order.
import { sprintf } from '@playfulsparkle/sprintf-js';
const firstName = "John";
const lastName = "Doe";
console.log(sprintf("Last name: %2$s, First name: %1$s", firstName, lastName));
// Output: Last name: Doe, First name: John
Named Placeholders
sprintf.js also supports named placeholders, making your format strings even more readable, especially when dealing with objects or complex data structures. You can define named arguments as an object and then refer to them in the format string using %(name)type
.
import { sprintf } from '@playfulsparkle/sprintf-js';
const user = { name: "Jane", age: 25 };
const message = sprintf("User %(name)s is %(age)d years old.", user);
console.log(message); // Output: User Jane is 25 years old.
const product = { id: "PROD123", price: 49.99 };
const productInfo = sprintf("Product ID: %(id)s, Price: $%.2f", product);
console.log(productInfo); // Output: Product ID: PROD123, Price: $49.99
Practical Applications of sprintf.js
sprintf.js can be incredibly useful in various JavaScript development scenarios:
Generating Dynamic URLs with Formatted Parameters
import { sprintf } from '@playfulsparkle/sprintf-js';
const baseUrl = "[https://api.example.com/users/%d](https://www.google.com/search?q=https://api.example.com/users/%25d)";
const userId = 123;
const apiUrl = sprintf(baseUrl, userId);
console.log(apiUrl); // Output: [https://api.example.com/users/123](https://www.google.com/search?q=https://api.example.com/users/123)
const searchUrl = "[https://search.example.com?query=%s&page=%d](https://www.google.com/search?q=https://search.example.com%3Fquery%3D%25s%26page%3D%25d)";
const query = "javascript";
const pageNumber = 2;
const fullSearchUrl = sprintf(searchUrl, encodeURIComponent(query), pageNumber);
console.log(fullSearchUrl); // Output: [https://search.example.com?query=javascript&page=2](https://www.google.com/search?q=https://search.example.com%3Fquery%3Djavascript%26page%3D2)
Creating Formatted Log Messages with Timestamps and Severity Levels
import { sprintf } from '@playfulsparkle/sprintf-js';
const timestamp = new Date().toISOString();
const level = "ERROR";
const message = "Failed to connect to database.";
const logMessage = sprintf("[%s] [%s] %s", timestamp, level, message);
console.log(logMessage);
// Example Output: [2025-03-27T00:19:50.000Z] [ERROR] Failed to connect to database.
Support and Compatibility
sprintf.js is designed to be highly compatible with various JavaScript environments. According to the GitHub repository:
- Node.js: Works seamlessly with modern Node.js versions.
- Browsers: Compatible with most modern web browsers. The repository provides a browser support table that you should refer to for specific details on supported versions. It's generally compatible with:
- Chrome
- Firefox
- Safari
- Edge
- Internet Explorer (check the table for specific supported versions)
For older browsers that might lack certain JavaScript features, you might need to include polyfills to ensure full compatibility. However, for most modern development environments, sprintf.js should work out of the box.
Conclusion
String formatting is a crucial skill for software developers, and the sprintf method offers a powerful and readable way to achieve precise control over text output. Playful Sparkle's sprintf.js library brings this widely adopted standard to the JavaScript world, providing a lightweight and efficient solution for developers who need robust string formatting capabilities. By mastering the format string placeholders and understanding the library's API, you can significantly improve the clarity and maintainability of your JavaScript code.
We encourage you to explore the sprintf.js library further on its GitHub repository: https://github.com/playfulsparkle/sprintf.js (opens in new window).
Questions
sprintf()
provides a more readable and controlled way to format strings compared to simple string concatenation. It offers features like padding, alignment, precision control, and named placeholders, making it easier to create complex and well-formatted output.
sprintf.js is a function (or a similar concept) used in many programming languages to format strings by embedding variables and applying specific formatting rules. It takes a format string containing placeholders and a list of arguments to be inserted into those placeholders.
Template literals (backticks ``
) are excellent for simple string interpolation. However, sprintf.js offers more advanced formatting options and better readability for complex formatting scenarios with multiple variables and specific formatting requirements.
Key features include a faithful implementation of the sprintf standard, lightweight nature, open-source license, support for various type specifiers, flexible argument order, and named placeholders.
sprintf.js is generally considered fast for most common use cases. Its lightweight nature helps minimize overhead. However, for extremely performance-critical applications with very high string formatting frequency, it's always recommended to benchmark and compare with other approaches.
The primary source of information is the official GitHub repository: https://github.com/playfulsparkle/sprintf.js (opens in new window). You can find detailed documentation, examples, and the source code there.
Yes, sprintf.js is designed to be compatible with both Node.js environments and modern web browsers. You can install it via NPM for Node.js projects and include it directly in your HTML for browser-based applications (or use a module bundler).
Let’s amplify your success together!
Request a Free QuoteRelated articles

A comprehensive guide to JSON-LD: Understanding structured data for the semantic web
Introduction In an increasingly interconnected digital world, data must be meaningful not only to humans but also to machines. Structured data, the backbone of the Semantic Web, enables this by allowing data to be easily interpreted and understood by search engines and other automated systems. Read moreabout A comprehensive guide to JSON-LD: Understanding structured data for the semantic web

A comprehensive guide to metadata: OpenGraph, Twitter, and Dublin Core
What is metadata and why is it important? In the digital era, metadata has become a foundational element in how we share, categorize, and discover content online. Read moreabout A comprehensive guide to metadata: OpenGraph, Twitter, and Dublin Core

How to choose the best image file format for your website
Choosing the right image file format for your website is critical for optimizing performance, enhancing user experience, and ensuring compatibility across devices and platforms. Read moreabout How to choose the best image file format for your website